What are Blueprints?
In Python Flask, a Blueprint is a way to organise your application into modules, which can have their own routes, static files, and templates. Each Blueprint functions like a mini application that can be registered with the main Flask application. This modular approach helps in organising code, reducing redundancy, and improving maintainability.
Analogy
Imagine that your Flask application is like a big public library. A library has different sections, such as fiction, non-fiction, science, history, etc. Each section has its own set of books, staff to manage the section, and layout. Although each section operates somewhat independently, they are all part of the same library.
In this analogy:
- The entire library is your main Flask application.
- Each section (fiction, non-fiction, etc.) is a Blueprint. Each section (Blueprint) has its own books (routes), staff (views and templates), and layout (static files and resources).
- The library’s management system that oversees all sections and ensures they work together seamlessly is akin to the main application that registers and coordinates the Blueprints.
Benefits of using blueprints
- Modularity: Code for different parts of your application can be split into separate Blueprints, making it easier to manage and understand.
- Reusability: Blueprints can be packaged and reused across different projects.
- Scalability: As your application grows, you can keep adding new Blueprints without cluttering your main application file.
- Separation of Concerns: Each Blueprint can encapsulate a specific feature or functionality of your application, promoting cleaner code architecture.
Blueprints in CKAN
In my CKAN extension (pigeon) example I will create a blueprint to display a new page using a new URL route.
When you create a new CKAN extension Blueprints are available by default, however, they are not activated. To activate blueprints you need to uncomment the “plugins.implements(plugins.IBlueprint)” line within the PigeonPlugin class in the plugin.py file plus you need to uncomment the “import ckanext.pigeons.views as views” line also.
The get_blueprint() method provides the Flask blueprint by calling get_blueprints() from the views module of the pigeon extension. This is essential for adding new routes and views to CKAN
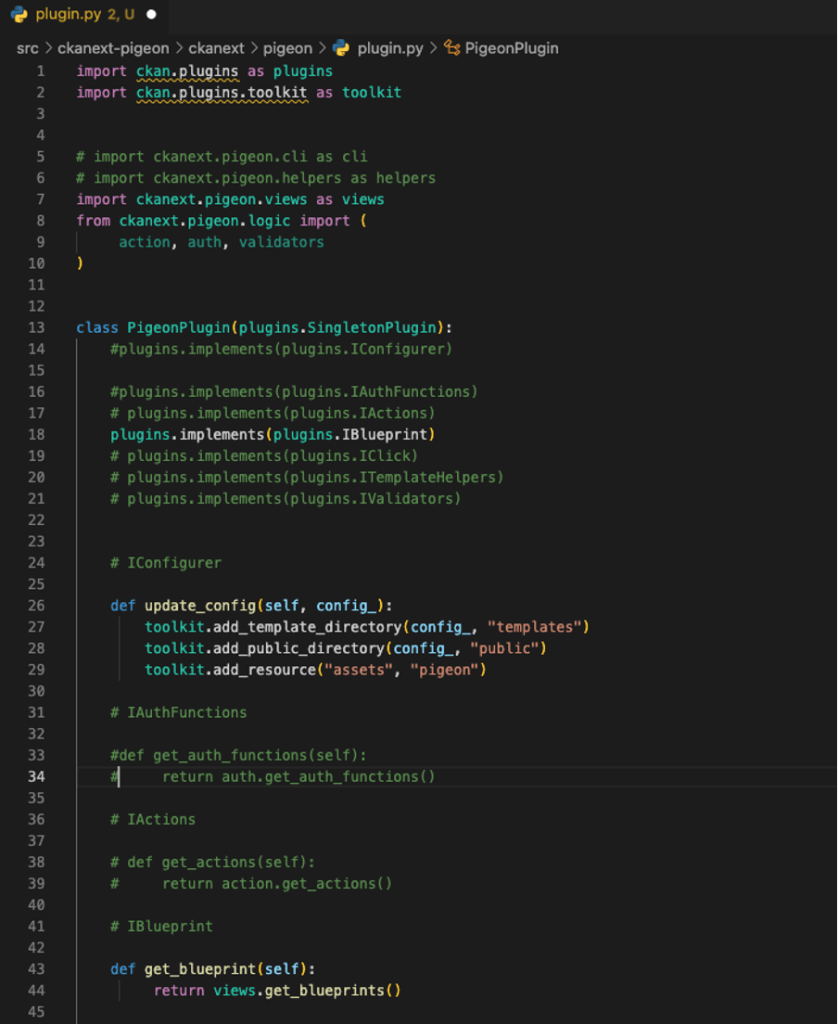
The main blueprint code (below) defines a Flask blueprint named “pigeon” and adds a single route to it.
Here’s a detailed summary:
- Import Blueprint: The Blueprint class is imported from the Flask package.
- Create Blueprint: A new blueprint named “pigeon” is created.
- Define Route Function: A function page is defined, which returns a specific string when called.
- Add URL Rule: The page function is linked to the URL path /pigeon/page within the blueprint.
- Return Blueprints: The get blueprints function is defined to return a list containing the pigeon blueprint.
In essence, this code sets up a reusable component (a blueprint) in a Flask application, which can be registered to a Flask app to handle requests to /pigeon/page.
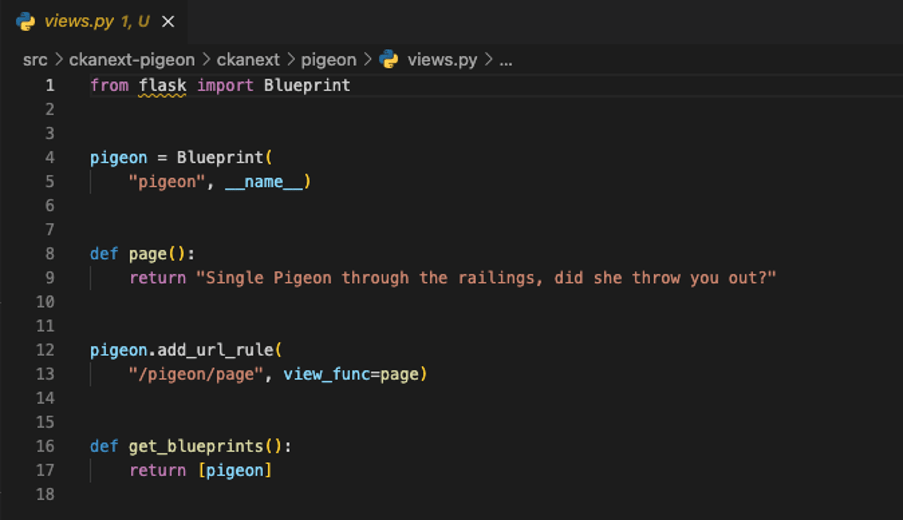
Now if we access the URL we see the following output:

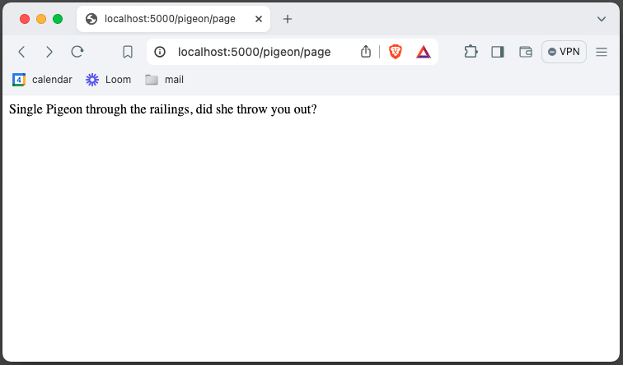
That’s all. You can also watch the video version of this article, CKAN Extensions: Creating a blueprint on the Link Digital YouTube channel.
Related articles
How to install CKAN using Docker Containers
How to install DataStore and DataPusher on CKAN
Create a CKAN development environment and install a new extension